Dangling Pointers in the Local Variables and Data Views
If you enable memory debugging, TotalView displays information in the Local Variables view about the variable’s memory status; that is, whether the memory is allocated or deallocated. The following small program allocates a memory block, sets a pointer to the start of the block, and then deallocates the block:
main(int argc, char **argv)
{
int *addr = 0; /* Pointer to start of block. */
addr = (int *) malloc (10 * sizeof(int));
/* Deallocate the block. addr is now dangling. */
free (addr);
/* Add some data to the array */
addr[0] = 1;
addr[1] = 2;
...
}
Figure 196 shows the Local Variables view. In the top view, execution was stopped before your program executed the
free() function. Note the memory indicator reporting that blocks are allocated.
After your program executes the free() function, the message changes to “Dangling.”
If you drag the variable from the Local Variables view to the Data View, it also displays the memory indicator:
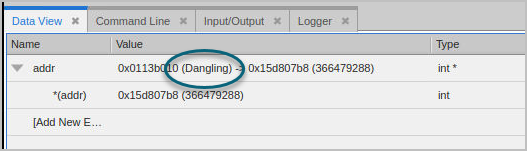